歡迎光臨重慶江北機械有限責任公司官網
關于我們
關于我們
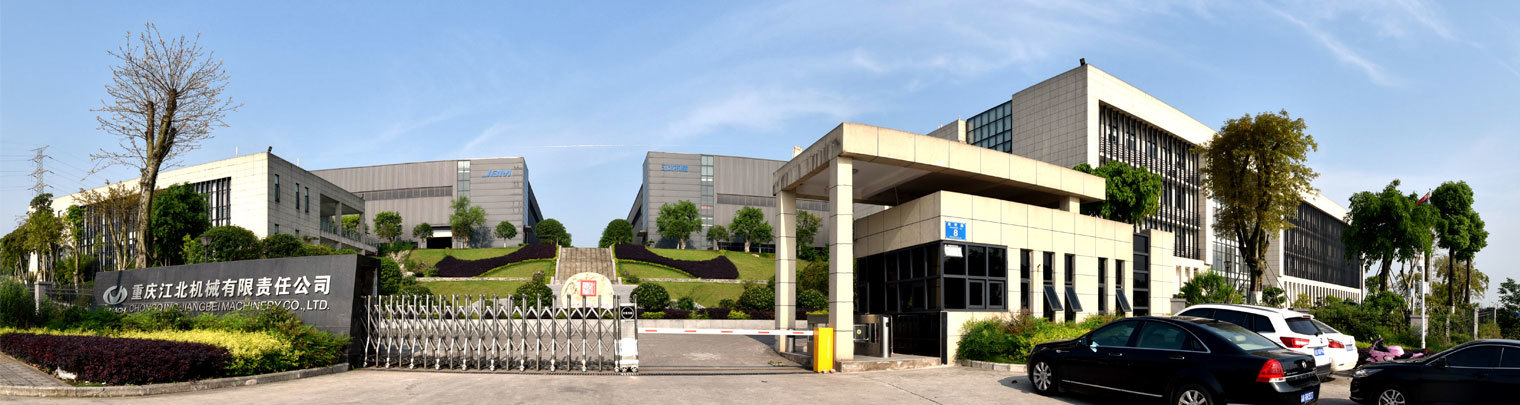
重慶江北機械有限責任公司(簡稱江北機械)
重慶江北機械有限責任公司
(簡稱江北機械)
始建于1941年,自1965年起專業生產制造分離機械產品,2016年實行混合所有制改革搬遷落戶至國家級開發區重慶兩江新區魚復工業園區,是工業和信息化部“專精特新小巨人”企業 ,高新技術企業,用戶5000余家遍及50多個國家和地區,是分離機械及其系統制造商、集成商和服務商。
江北機械是中國通用機械工業協會副會長單位、中通協分離機械分會理事長單位、全國分離機械標準化技術委員會副主任委員單位、中國石化聯合會過濾與分離專委會副主任委員單位、中國無機鹽工業協會磷酸鐵鋰專委會副主任委員單位、中國淀粉工業協會理事單位。
更多詳細內容 >>
公司成立
產品遠銷50多個國家和地區
近三年銷售的高新產品
授權有效專利146項
服務客戶5000余家
公司成立
產品遠銷50多個國家和地區
近三年銷售的高新產品1000余臺套
授權有效專利146項
服務客戶5000余家
產品推薦
產品推薦
新聞資訊
新聞資訊
評優創新,共話未來│ 江機公司2024年四季度管理論壇圓滿落幕
2024/11/30
MORE+
第十二屆中國(上海)國際流體機械展覽圓滿落幕 | 重慶江北機械感謝您一路相伴!
2024/11/28
MORE+
真誠服務,共贏未來|重慶江北機械2024年秋季離心機培訓會圓滿舉辦!
2024/11/01
MORE+